Viem: The ultimate web3 library

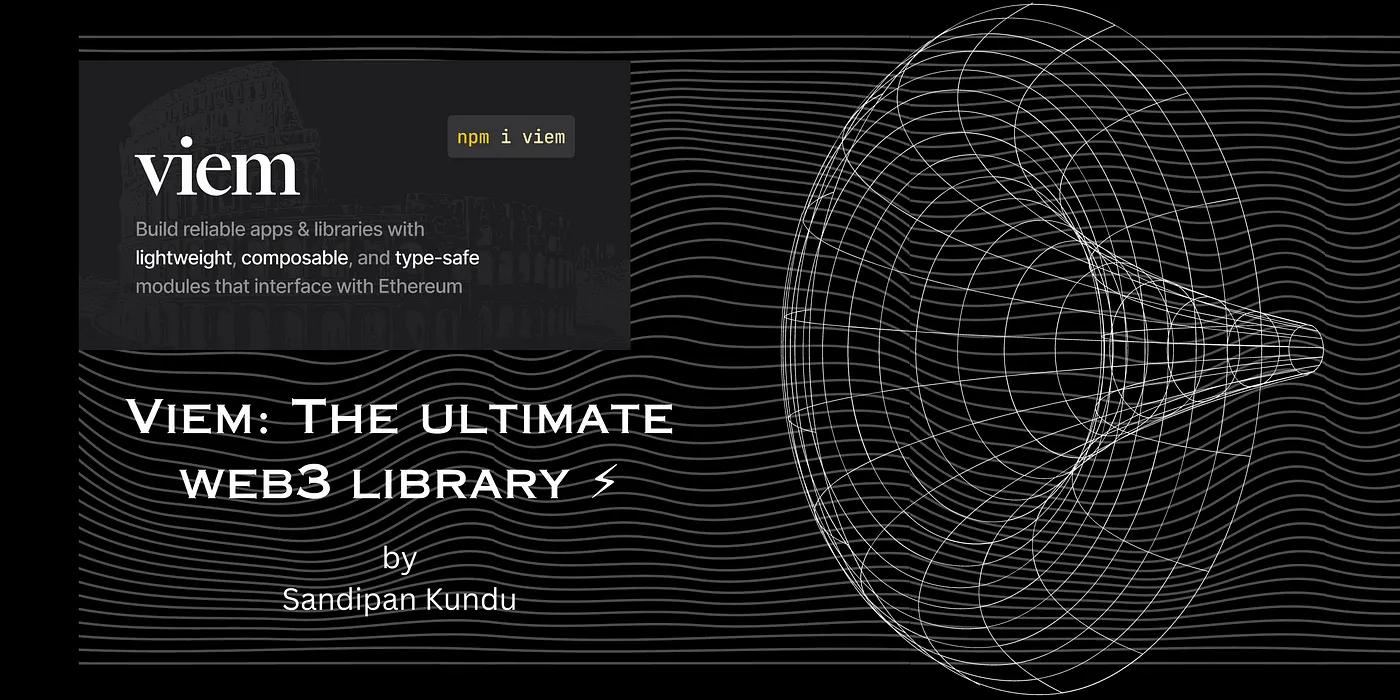

Viem is a TypeScript library for interacting with EVM blockchains. It is a modern, lightweight, and easy-to-use library that is designed to make building web3 dapps easy and blazing fast!
Viem is a relatively new library, but it has a number of advantages over ethers.js and web3.js.
- TypeScript support: Viem is written in TypeScript, which makes it more type-safe than ethers.js and web3.js. This can help to prevent errors and make your code more readable.
- Concise API: Viem’s API is more concise than ethers.js and web3.js. This makes it easier to learn and use, especially for beginners.
- Bundle size and Performance: Viem adopts a minimalist modular architecture to achieve a bundle size of 35kb — significantly lower than other libraries. Viem further tunes performance by only executing heavy asynchronous tasks when required and optimized encoding/parsing algorithms.
In this blog post, we will cover how to install and implement viem in our web3 applications.
Installing viem
Viem is available as a npm package. To install viem, run the following command in your terminal:
npm install viem
viem uses the concept of ‘clients’ similar to ethers js ‘providers’ to connect to a blockchain.
- A Public Client which provides access to Public Actions, such as
getBlockNumber
andgetBalance
. - A Wallet Client which provides access to Wallet Actions, such as
sendTransaction
andsignMessage
.
So, let’s start by declaring our Client and Transport. For this example, we will use the HTTP transport for the Shardeum Sphinx chain. You can view the list of supported chains here, but also you can connect with unsupported chains manually.
import { createPublicClient, http } from'viem'import {shardeumSphinx } from'viem/chains'exportconst client = createPublicClient({chain: shardeumSphinx,transport: http(),})
Now that you have a Client set up, you can now interact with the blockchain using the respective RPC methods. We will discuss some of the most commonly used methods.
Reading data
Using the getBalance() API which returns the balance of an address in wei.
const balance = await client.getBalance({address: '0x24DC7bfFaf068051946521c5A712E259acbCC6f4',})console.log(balance);
Sending a transaction
Using the sendTransaction() API to send native tokens from one wallet to another. Note, that for this, we need to configure the walletClient also.
import { createPublicClient, createWalletClient, http } from'viem'import {shardeumSphinx } from'viem/chains'export constclient = createPublicClient({chain: shardeumSphinx,transport: http(),})export constwalletClient = createWalletClient({chain: shardeumSphinx,transport: http(),})consthash = await walletClient.sendTransaction({account:'0x68fCFF447Ee091Ea4b0D1EB1F9b64d65cDbfdc8a',to: '0x24DC7bfFaf068051946521c5A712E259acbCC6f4',value: 10000000000000000000n //10 SHM})
Interacting with Smart Contracts
Let’s deploy a basic solidity smart contract:
pragma solidity ^0.8.4;contract Counter {// This is a public variable that can be accessed from other contracts.uint public counter;// This is a constructor function that is called when the contract is created.constructor() {counter = 0;}// This is a public function that can be called from other contracts.function increment() public {counter += 1;}// This is a public function that can be called from other contracts.function getCounter() public view returns (uint) {return counter;}}
You can also use the contract address directly deployed on Shardeum Sphinx testnet: 0xCA9044e7f0987Ca5562C66251234feF7E453C143
import { createPublicClient, createWalletClient, http} from 'viem'import {shardeumSphinx } from 'viem/chains'import { Abi } from './abi'export const client = createPublicClient({ chain: shardeumSphinx, transport: http(),})export const walletClient = createWalletClient({ chain: shardeumSphinx, transport: http(),})const data = await client.readContract({ address: '0xCA9044e7f0987Ca5562C66251234feF7E453C143', abi: Abi, functionName: 'getCounter',})console.log(data);
Next, try using the writeContract() API to increment counter by 1.
And that’s it!! 🎉
You have now learned how to install, configure and implement viem in your web3 applications. Personally I am very bullish on this project and planning to continue using it in my applications.
Please refer to the full documentation here to learn more functionalities.